Especially when working with BLoCs in Flutter, it becomes a regular habit to create a new instance of a state based on a previous state. At least when working with Equatable which is used to reduce the number of state emissions and thus the number of UI repaints when nothing has actually changed.
But independent of the BLoC pattern or the bloc library there can be several other situations in which you want to clone an object, giving the clone other properties than the original object.
Let’s analyze what possibilities we get from Dart and then evaluate what we can use.
There have been discussions about giving Dart the functionality of cloning (or rather shallow copying) natively. However, it seemed like the consensus was that this should not be possible from the outside but rather be provided by the object itself.
It means that every class whose object instances should be copyable or clonable at runtime, need to have respective methods with custom logic that is to be defined by you.
But what should such a method look like? What requirements should it fulfill? Let’s list the requirements and write tests based on them. Then we test different approaches with respect to these requirements:
- When comparing the original object and the clone via “==”, it should evaluate to false. Otherwise we would have just copied the reference rather than the value
- This is an implicit consequence of the above but I list it here separately: changing a property in the clone does not in any way affect the original’s property of that kind
- Given a null value as an argument of the clone method, the cloned object should be null. This seems clear but you will see while I listed this
- Omitting a property as an argument of the clone method results in this property having the same value as the original’s property of that kind
Let’s define our test object like this:
1class SampleModel {
2 SampleModel({
3 this.id,
4 this.header,
5 this.body,
6 });
7
8 int id;
9 String header;
10 String body;
11}
Okay now that we have a structure we can run tests on, let’s define the test cases we will apply to different copy algorithms:
- “Values are copied instead of instances”: we use the copy algorithm to clone an instance of our model. We then check equality via “==” and assume it’s false”
- “Null values override”: when setting a value to null during copy it should be null afterwards
- “Omitted values don’t change”: when omitting a value during copy, it should have the same value as the original object
Testing different approaches
Our aim is to find a method to clone objects that fulfills the above requirements. Let’ test different approaches regarding these requirements.
Simple assignment
Now we transform the test cases to actual unit tests and test the first copy algorithm – a simple assignment:
1group('Assignment', () {
2 test('Instances are different', () {
3 SampleModel modelA = _createSampleInstance();
4
5 SampleModel modelB = modelA;
6 modelB.header = "Model B";
7
8 _assertNonEquality(modelA, modelB);
9 });
10
11 test('Null values override existing values', () {
12 SampleModel modelA = _createSampleInstance();
13
14 SampleModel modelB = modelA;
15 modelB.header = null;
16
17 _assertThatNullValuesOverride(modelA, modelB);
18 });
19
20 test('Omitted values override existing values', () {
21 SampleModel modelA = _createSampleInstance();
22
23 SampleModel modelB = modelA;
24 modelB.id = 3;
25 modelB.body = 'Testbody';
26
27 _assertThatOmittedValuesHaveOriginalValues(modelA, modelB);
28 });
29 });
30}
31
32SampleModel _createSampleInstance() {
33 SampleModel modelA = SampleModel(
34 id: 1,
35 header: 'Model A',
36 body: 'This is a body'
37 );
38 return modelA;
39}
40
41void _assertNonEquality(SampleModel modelA, SampleModel modelB) {
42 expect(modelA == modelB, false);
43}
44
45void _assertThatNullValuesOverride(SampleModel modelA, SampleModel modelB) {
46 expect(modelB.header == null, true);
47}
48
49void _assertThatOmittedValuesHaveOriginalValues(SampleModel modelA, SampleModel modelB) {
50 expect(modelA.header == modelB.header, true);
51}
These are the test results:
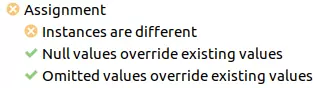
The first tests fails because by assigning modelA
to modelB
only copies the reference, not the values.
The value we set to null is null in modelB
and the other values we did not set have the original value. However, we don’t want the original object to change, when we change a property.
By the way: if we work with classes that extend Equatable
, we would have another problem: since it’s annotated @immutable
, the above code would not even compile.
Creating a new instance
If assigning the original object to a new variable just copies the reference, then let’s improve it by creating a completely new object and assigning all properties we want to change compared to the original object:
1group('Manually creating a new instance', () {
2 test('Instances are different', () {
3 SampleModel modelA = _createSampleInstance();
4
5 SampleModel modelB = SampleModel(
6 id: modelA.id,
7 header: modelA.header,
8 body: modelA.body
9 );
10
11 _assertNonEquality(modelA, modelB);
12 });
13
14 test('Null values override existing values', () {
15 SampleModel modelA = _createSampleInstance();
16
17 SampleModel modelB = SampleModel(
18 header: null
19 );
20
21 _assertThatNullValuesOverride(modelA, modelB);
22 });
23
24 test('Omitted values override existing values', () {
25 SampleModel modelA = _createSampleInstance();
26
27 SampleModel modelB = SampleModel(
28 id: 3, body: 'Testbody'
29 );
30
31 _assertThatOmittedValuesHaveOriginalValues(modelA, modelB);
32 });
33 });
These are the results of this approach:
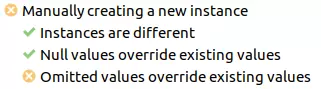
We fixed an issue of the previous approach: we end up with a different instance of the same class.
The problem we have now: all the properties we don’t provide are set to null. We would have to manually set every single property we want to apply from the original instance. If we had an object with 5 or more properties, this can be annoying already. We need to find a way to let the algorithm be aware of the original properties while not using reference copy.
Simple “copy” method
Let’s extend the model with a copyWith
method. This is actually a common approach when working with TextTheme
s.
1SampleModel copyWith({
2 int id, String header, String body
3}) => SampleModel(
4 id: id ?? this.id,
5 header: header ?? this.header,
6 body: body ?? this.body
7);
Okay, let’s test it:
1group('Simple copyWith', () {
2 test('Instances are different', () {
3 SampleModel modelA = _createSampleInstance();
4
5 SampleModel modelB = modelA.copyWith();
6
7 _assertNonEquality(modelA, modelB);
8 });
9
10 test('Null values override existing values', () {
11 SampleModel modelA = _createSampleInstance();
12
13 SampleModel modelB = modelA.copyWith(header: null);
14
15 _assertThatNullValuesOverride(modelA, modelB);
16 });
17
18 test('Omitted values override existing values', () {
19 SampleModel modelA = _createSampleInstance();
20
21 SampleModel modelB = modelA.copyWith(
22 id: 3, body: 'Testbody'
23 );
24
25 _assertThatOmittedValuesHaveOriginalValues(modelA, modelB);
26 });
27 });
The test results:
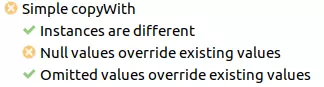
Our issue here is that Dart is unable to differentiate between omitted arguments and actual null values.
Copy method using Nullables
In order to let the method be aware of the given values being null, we create a wrapper around a given type. This gives us the ability to intentionally set a value to null even if it’s a primitive type.
1class Nullable<T> {
2 T _value;
3
4 Nullable(this._value);
5
6 T get value {
7 return _value;
8 }
9}
Our new method now expects Nullables instead of the actual types:
1SampleModel copyWithImproved({
2 Nullable<int> id, Nullable<String> header, Nullable<String> body
3}) => SampleModel(
4 id: id == null ? this.id : id.value == null ? null : id.value,
5 header: header == null ? this.header : header.value == null ? null : header.value,
6 body: body == null ? this.body : body.value == null ? null : body.value,
7);
Let’s adapt the tests to test our new method:
1group('copyWith using Nullable', () {
2 test('Instances are different', () {
3 SampleModel modelA = _createSampleInstance();
4
5 SampleModel modelB = modelA.copyWithImproved();
6
7 _assertNonEquality(modelA, modelB);
8 });
9
10 test('Null values override existing values', () {
11 SampleModel modelA = _createSampleInstance();
12
13 SampleModel modelB = modelA.copyWithImproved(
14 header: Nullable(null)
15 );
16
17 _assertThatNullValuesOverride(modelA, modelB);
18 });
19
20 test('Omitted values override existing values', () {
21 SampleModel modelA = _createSampleInstance();
22
23 SampleModel modelB = modelA.copyWithImproved(
24 id: Nullable(3), body: Nullable('Tesbody')
25 );
26
27 _assertThatOmittedValuesHaveOriginalValues(modelA, modelB);
28 });
29});
When the given arguments are null, we take the values of the instance. If not, we take the value of the Nullable.
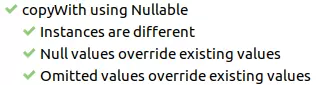
Conclusion
If we want a method that copies a given object without changing the original object and only want to provide those arguments that should be changed compared to the original, we can make use of a wrapper around primitive types. There is no native generic way to copy objects so it’s necessary to implement a custom method every time new.
dut
Marc
In reply to dut's comment
Juan Julio