Especially when dragging markers across an image, it’s quite common to show a magnifier that enlarges the area because it’s covered by the finger. Let’s have a look at how to implement that in Flutter.
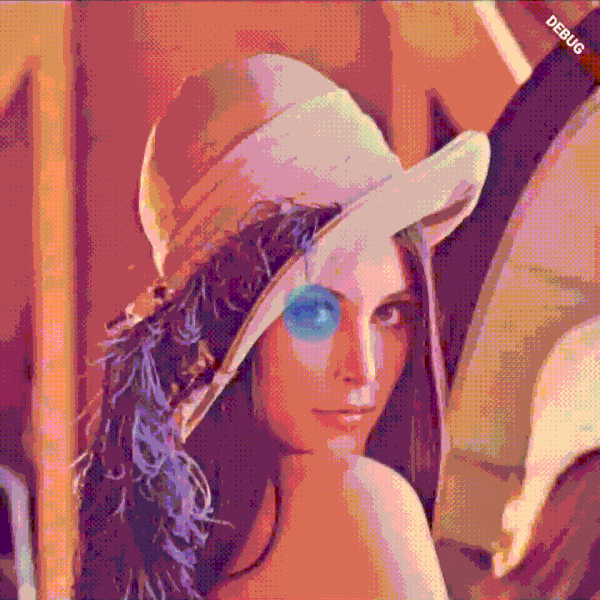
Our goal
This above description is very broad. Let’s go into further detail by defining the key features of our magnifier:
- There is a half-transparent circle than can be dragged across the image
- Once it’s being dragged, the magnifier appears on the top left
- Whenever the circle interferes with the magnifier, it jumps to the top right
- The magnifier enlarges the area of the underlying image by a factor that is definable
- Once the drag ends, the magnifier disappears
- This does not only work for images but for every widget
Implementation
We start by implementing a sample screen that contains the image we want to add the possibility to zoom on. For this, we use the most popular test image: Lena Forsén, better known as Lenna.
1@override
2 Widget build(BuildContext context) {
3 return Stack(
4 children: [
5 Image(
6 image: AssetImage('assets/lenna.png')
7 )
8 ],
9 );
10 }
We use a Stack
widget because we want to put the touch bubble and the magnifier on top later on.
The touch bubble
Now we need the touch bubble the user should be able to position on the part of the image that should be magnified.
1class _SampleImageState extends State<SampleImage> {
2 static const double touchBubbleSize = 20;
3
4 Offset position;
5 double currentBubbleSize;
6 bool magnifierVisible = false;
7
8 @override
9 void initState() {
10 currentBubbleSize = touchBubbleSize;
11 SystemChrome.setEnabledSystemUIOverlays([SystemUiOverlay.bottom]);
12 super.initState();
13 }
14
15 @override
16 Widget build(BuildContext context) {
17 return Stack(
18 children: [
19 Magnifier(
20 position: position,
21 visible: magnifierVisible,
22 child: Image(
23 image: AssetImage('assets/lenna.png')
24 ),
25 ),
26 _getTouchBubble()
27 ],
28 );
29 }
30
31 Positioned _getTouchBubble() {
32 return Positioned(
33 top: position == null ? 0 : position.dy - currentBubbleSize / 2,
34 left: position == null ? 0 : position.dx - currentBubbleSize / 2,
35 child: GestureDetector(
36 child: Container(
37 width: currentBubbleSize,
38 height: currentBubbleSize,
39 decoration: BoxDecoration(
40 shape: BoxShape.circle,
41 color: Theme.of(context).accentColor.withOpacity(0.5)
42 ),
43 )
44 )
45 );
46 }
We need to store the position of the touch bubble so that we can provide that piece of information to the magnifier. This is necessary so that the magnifier can only enlarge the particular area that is determined by the touch bubble.
We also have a static size of the touch bubble which determines the base size the bubble returns to when no interaction is happening. We also need the actual current size that can differ when the user drags the bubble. To highlight the drag event we want the bubble to grow by 50 %.
We could implement it like above and just extract the visual description of the touch bubble into its own method which resides in the sample image widget. However, it’s a good idea to put it into its own widget.
This will make it look like this:
1import 'package:flutter/material.dart';
2
3class TouchBubble extends StatelessWidget {
4 TouchBubble({
5 @required this.position,
6 this.bubbleSize
7 });
8
9 final Offset position;
10 final double bubbleSize;
11
12 @override
13 Widget build(BuildContext context) {
14 return Positioned(
15 top: position == null ? 0 : position.dy - bubbleSize / 2,
16 left: position == null ? 0 : position.dx - bubbleSize / 2,
17 child: GestureDetector(
18 child: Container(
19 width: bubbleSize,
20 height: bubbleSize,
21 decoration: BoxDecoration(
22 shape: BoxShape.circle,
23 color: Theme.of(context).accentColor.withOpacity(0.5)
24 ),
25 )
26 )
27 );
28 }
29}
So far so good. But yet, there is no interaction possible:
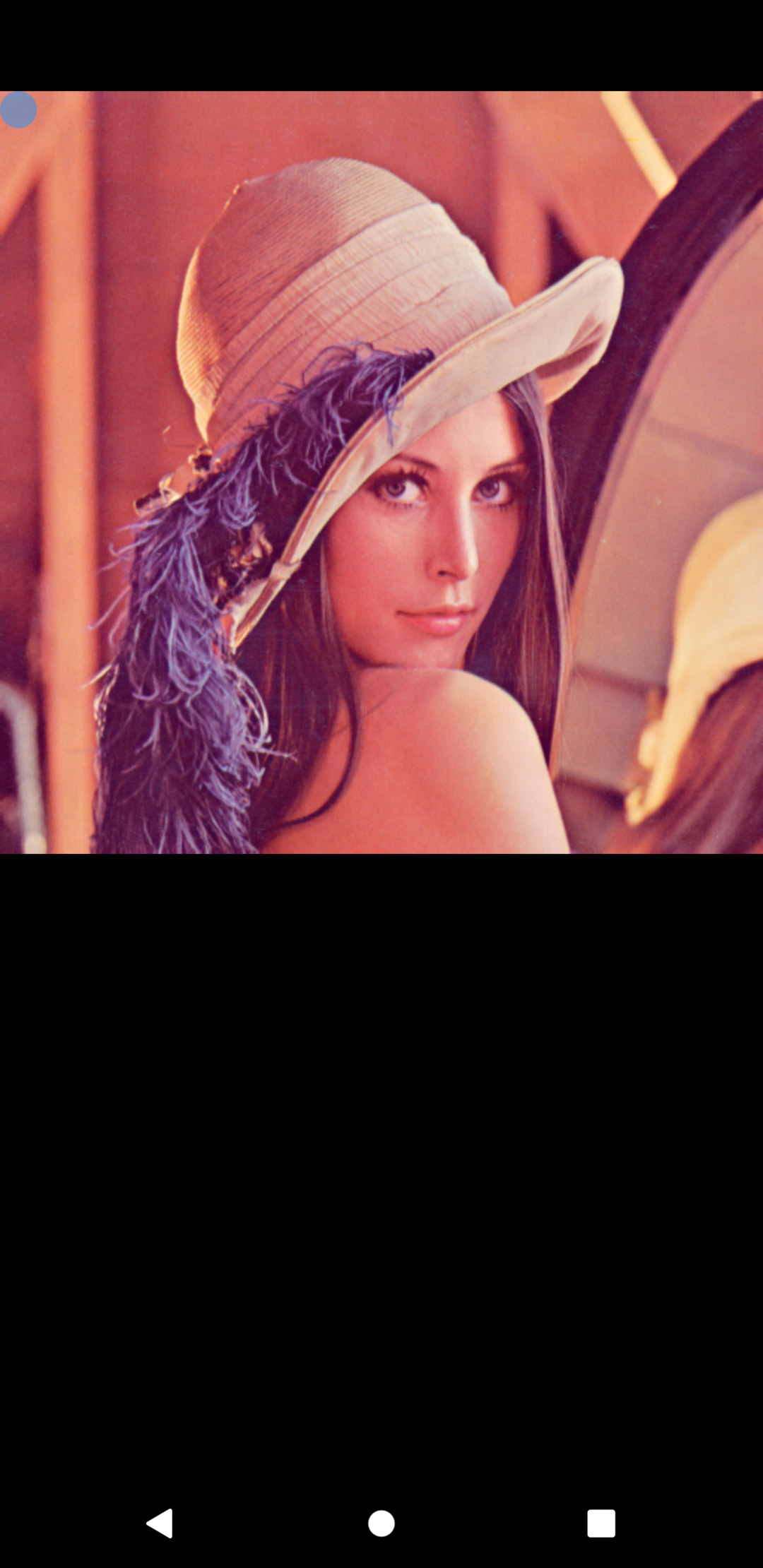
The touch bubble is visible at the top left because that is the fallback value for the top
and left
property of the Positioned
widget inside our TouchBubble
class.
In order to add interactivity to our magnifier, we let the TouchBubble
expect some callbacks in its constructor:
1import 'package:flutter/material.dart';
2
3class TouchBubble extends StatelessWidget {
4 TouchBubble({
5 this.position,
6 @required this.onStartDragging,
7 @required this.onDrag,
8 @required this.onEndDragging,
9 @required this.bubbleSize,
10 }) : assert(onStartDragging != null),
11 assert(onDrag != null),
12 assert(onEndDragging != null),
13 assert(bubbleSize != null && bubbleSize > 0);
14
15 final Offset position;
16 final double bubbleSize;
17 final Function onStartDragging;
18 final Function onDrag;
19 final Function onEndDragging;
20
21 @override
22 Widget build(BuildContext context) {
23 return Positioned(
24 ...
25 child: GestureDetector(
26 onPanStart: (details) => onStartDragging(details.globalPosition),
27 onPanUpdate: (details) => onDrag(details.globalPosition),
28 onPanEnd: (_) => onEndDragging(),
29 ...
30 )
31 );
32 }
33}
Now we have three new additional arguments: onStartDragging
, onDrag
and onEndDragging
. The behavior that is triggered by these functions, will be defined in the parent widget. We annotate all of them (expect for position
because we have a fallback) as @required
which tells our IDE to warn us if we omit this argument. Additionally, we provide asserts for runtime errors to occur at the correct place if we ignore the suggestions of our IDE.
onStartDragging
and onDrag
are expected to be provided with the new absolute (global) position. onEndDragging
does not expect any argument.
Our new SampleImage
widget looks like this:
1import 'package:flutter/material.dart';
2import 'package:flutter/services.dart';
3import 'package:flutter_magnifier/touch_bubble.dart';
4
5class SampleImage extends StatefulWidget {
6 @override
7 _SampleImageState createState() => _SampleImageState();
8}
9
10class _SampleImageState extends State<SampleImage> {
11 static const double touchBubbleSize = 20;
12
13 Offset position;
14 double currentBubbleSize;
15 bool magnifierVisible = false;
16
17 @override
18 void initState() {
19 currentBubbleSize = touchBubbleSize;
20 SystemChrome.setEnabledSystemUIOverlays([SystemUiOverlay.bottom]);
21 super.initState();
22 }
23
24 @override
25 Widget build(BuildContext context) {
26 return Stack(
27 children: [
28 Image(
29 image: AssetImage('assets/lenna.png')
30 ),
31 TouchBubble(
32 position: position,
33 bubbleSize: currentBubbleSize,
34 onStartDragging: _startDragging,
35 onDrag: _drag,
36 onEndDragging: _endDragging,
37 )
38 ],
39 );
40 }
41
42 void _startDragging(Offset newPosition) {
43 setState(() {
44 magnifierVisible = true;
45 position = newPosition;
46 currentBubbleSize = touchBubbleSize * 1.5;
47 });
48 }
49
50 void _drag(Offset newPosition) {
51 setState(() {
52 position = newPosition;
53 });
54 }
55
56 void _endDragging() {
57 setState(() {
58 currentBubbleSize = touchBubbleSize;
59 magnifierVisible = false;
60 });
61 }
62}
The position is updated on drag start and on drag update. When the dragging starts, the mag is made visible and increases its size by 50 %. When dragging has ended, the size is turned back to normal and the magnifier is turned invisible.
It leads to a UI that behaves like this:
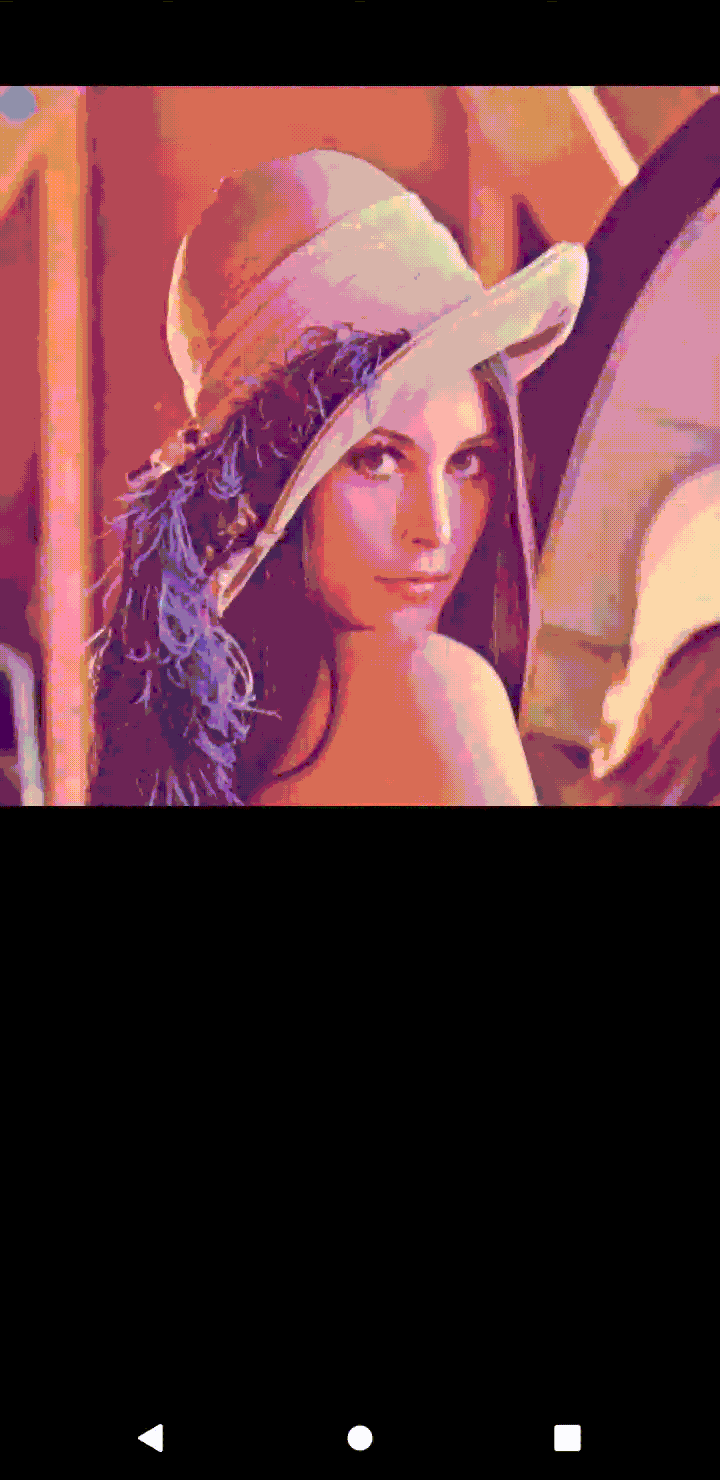
The magnifier
Before we jump into the implementation, let’s think for a second, what magnifying actually means. We take an image, scale it up, move the image to where the center of the mag is and then crop it so that only the inner part of the mag shows the translated image.
The translation is also influenced by the scale factor. Visually this can be explained like this:
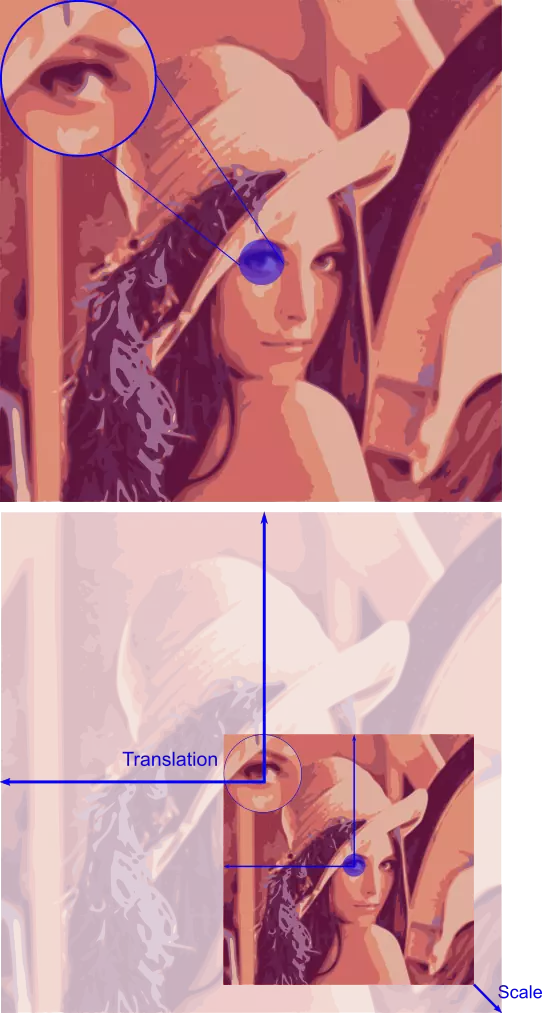
Let’s first create a new widget that represents our magnifier:
1class Magnifier extends StatefulWidget {
2 const Magnifier({
3 @required this.child,
4 @required this.position,
5 this.visible = true,
6 this.scale = 1.5,
7 this.size = const Size(160, 160)
8 }) : assert(child != null);
9
10 final Widget child;
11 final Offset position;
12 final bool visible;
13 final double scale;
14 final Size size;
15
16 @override
17 _MagnifierState createState() => _MagnifierState();
18}
19
20class _MagnifierState extends State<Magnifier> {
21 Size _magnifierSize;
22 double _scale;
23 Matrix4 _matrix;
24
25 @override
26 void initState() {
27 _magnifierSize = widget.size;
28 _scale = widget.scale;
29 _calculateMatrix();
30
31 super.initState();
32 }
33
34 @override
35 void didUpdateWidget(Magnifier oldWidget) {
36 super.didUpdateWidget(oldWidget);
37
38 _calculateMatrix();
39 }
40
41 @override
42 Widget build(BuildContext context) {
43 return Stack(
44 children: [
45 widget.child,
46 if (widget.visible && widget.position != null)
47 _getMagnifier(context)
48 ],
49 );
50 }
51
52 void _calculateMatrix() {
53 if (widget.position == null) {
54 return;
55 }
56
57 setState(() {
58 double newX = widget.position.dx - (_magnifierSize.width / 2 / _scale);
59 double newY = widget.position.dy - (_magnifierSize.height / 2 / _scale);
60
61 final Matrix4 updatedMatrix = Matrix4.identity()
62 ..scale(_scale, _scale)
63 ..translate(-newX, -newY);
64
65 _matrix = updatedMatrix;
66 });
67 }
68}
We have a few constructor arguments here. The most relevant are child
and position
. Because we want this feature to work with every widget, we need this widget to be provided (child
parameter). In order to determine which part of the underlying widget should be magnified, we also let it require a position
parameter.
visible
is used to only display it when the user drags the bubble. scale
and size
should be self-explanatory and have a default value.
The crucial business logic here is the _calculateMatrix()
method because it determines scaling and translation based on the position of the touch bubble. We take the identity matrix and use the scale and translation method to update it. Important: we need to use the center of the touch bubble as the anchor. That’s why we subtract half of the height and the width to determine the position.
Okay, now the part of drawing the actual magnifier is still missing. We call _getMagnifier()
but yet, there is no implementation.
1Widget _getMagnifier(BuildContext context) {
2 return Align(
3 alignment: Alignment.topLeft,
4 child: ClipOval(
5 child: BackdropFilter(
6 filter: ImageFilter.matrix(_matrix.storage),
7 child: Container(
8 width: _magnifierSize.width,
9 height: _magnifierSize.height,
10 decoration: BoxDecoration(
11 color: Colors.transparent,
12 shape: BoxShape.circle,
13 border: Border.all(color: Colors.blue, width: 2)
14 ),
15 ),
16 ),
17 );
18}
This deserves some explanation. Now, the crucial widget here is BackdropFilter. This filter takes a filter and a child. It applies the given filter to the child. The filter we use is matrix
. This lets us apply the calculated matrix to the child. It’s very important to wrap it in a ClipOval because we only want a sized circle to be seen and not the whole scaled and translated duplication of our original image.
In order to improve the possibility to place the bubble exactly where we want to, we can add a crosshair to the magnifier. This requires us to replace the Container
by a self-drawn widget.
1import 'package:flutter/material.dart';
2
3class MagnifierPainter extends CustomPainter {
4 const MagnifierPainter({
5 @required this.color,
6 this.strokeWidth = 5
7 });
8
9 final double strokeWidth;
10 final Color color;
11
12 @override
13 void paint(Canvas canvas, Size size) {
14 _drawCircle(canvas, size);
15 _drawCrosshair(canvas, size);
16 }
17
18 void _drawCircle(Canvas canvas, Size size) {
19 Paint paintObject = Paint()
20 ..style = PaintingStyle.stroke
21 ..strokeWidth = strokeWidth
22 ..color = color;
23
24 canvas.drawCircle(
25 size.center(
26 Offset(0, 0)
27 ),
28 size.longestSide / 2, paintObject
29 );
30 }
31
32 void _drawCrosshair(Canvas canvas, Size size) {
33 Paint crossPaint = Paint()
34 ..strokeWidth = strokeWidth / 2
35 ..color = color;
36
37 double crossSize = size.longestSide * 0.04;
38
39 canvas.drawLine(
40 size.center(Offset(-crossSize, -crossSize)),
41 size.center(Offset(crossSize, crossSize)),
42 crossPaint
43 );
44
45 canvas.drawLine(
46 size.center(Offset(crossSize, -crossSize)),
47 size.center(Offset(-crossSize, crossSize)),
48 crossPaint
49 );
50 }
51
52 @override
53 bool shouldRepaint(CustomPainter oldDelegate) {
54 return true;
55 }
56}
We start by drawing a circle of the given size but in stroke mode so we only draw the outline. After that, we draw the cross by using the center and shifting it by 4 % of the magnifier size to the top left and bottom right.
Now the _getMagnifier()
method in the Magnifier
class looks like this:
1Widget _getMagnifier(BuildContext context) {
2 return Align(
3 alignment: Alignment.topLeft,
4 child: ClipOval(
5 child: BackdropFilter(
6 filter: ImageFilter.matrix(_matrix.storage),
7 child: CustomPaint(
8 painter: MagnifierPainter(
9 color: Theme.of(context).accentColor
10 ),
11 size: _magnifierSize,
12 ),
13 ),
14 ),
15 );
16 }
What we want to take care of now is that the magnifier jumps to the top right when our touch bubble’s position interferes with the magnifier.
1Alignment _getAlignment() {
2 if (_bubbleCrossesMagnifier()) {
3 return Alignment.topRight;
4 }
5
6 return Alignment.topLeft;
7 }
8
9 bool _bubbleCrossesMagnifier() => widget.position.dx < widget.size.width &&
10 widget.position.dy < widget.size.height;
Instead of assigning Alignment.topLeft
statically to the magnifier, we decide whether to use topLeft
or topRight
based on its position.
Now we need to add an extra block to the calculateMatrix()
method:
1if (_bubbleCrossesMagnifier()) {
2 final box = context.findRenderObject() as RenderBox;
3 newX -= ((box.size.width - _magnifierSize.width) / _scale);
4}
Whenever the conditions are met, we influence the matrix translation by shifting the newX
by the whole width subtracted by the mag width because that is exactly the amount by which the magnifier moves when the alignment is changed from left to right.
Closing comment
Using the expensive but handy Widget BackdropFilter
and a little bit of matrix calculation, we were able to implement a magnifier that is able to enlarge every child widget given a position. The magnifier is located at the top left but jumps out of the way when our touch collides with the position of the magnifier.
You can see it in action (and see the code) by having a look at my edge detection project. The magnifier is used on touch bubbles that mimic the detected edges around a dynamic image.
Arthur Miranda
Marc
In reply to Arthur Miranda's comment
Marc
Tanakit
Marc
In reply to Tanakit's comment
Tanakit
In reply to Marc's comment
Mohammed